Below I will highlight a quick and simple way to set up a conditional breakpoint in VS.NET. This breakpoint will only be hit once the condition we set is = 'True'.
This method of setting up a conditional breakpoint can be quite useful when looping through a large list of values, and the developer wants to only debug the code once a certain value has arisen.
In the code below, I created a simple ArrayList and added some characters to it (A-G). Now let's say that I really only want to debug once the variable 'alItem = "D"'. Well I could place a breakpoint on the line that reads 'MyValue = alItem' and keep pressing 'F5' on the keyboard until the value of 'alitem = "D"', or I can set up a conditional breakpoint, so execution only stops once the value I want is set.
To begin, let's take a look at the code for this example below:
Dim al As New ArrayList
al.Add("A")
al.Add("B")
al.Add("C")
al.Add("D")
al.Add("E")
al.Add("F")
al.Add("G")
Dim MyValue As String = String.Empty
For Each alItem As String In al
MyValue = alItem
Next
1st, place a breakpoint on the line in which we want to set up a conditional breakpoint as pictured below:

2nd, open the 'Breakpoints' window by going to 'Debug -> Windows -> Breakpoints' as shown below:

3rd, right click the 'Condition' column description for the breakpoint set and select 'Condition' as shown below. This is where we dictate what condition we want to break execution:
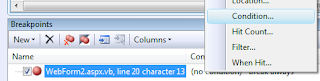
4th, enter in the condition we want to break on debugging; in our case I entered 'alItem = "D"' and made sure the 'Is True' radio button is selected:
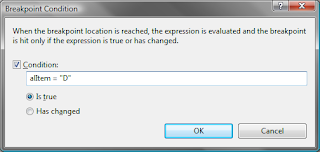
Lastly, run the code. You will notice that the code will only break execution when the condition we set up is 'True' as pictured below.

That's it! Hopefully this will save you a little time debugging in the future.