Recently while using VS.NET 2010 I had an unsettling feeling when I opened my solution file (.sln) that I have been working on for the past 3 years to find that only 1 of the 3 associated projects opened up. Well I immediately knew everything was ok because all of the directories, etc. still existed for the unopened projects, so I had to figure out how to fix the problem.
This particular problem occurred due to a corrupted .sln file. The .sln file associated with a VS.NET solution contains the meta data in text format of the associated project and source control information. To see this information, navigate to the .sln file in Windows Explorer and right click to select 'Open with' and select either Notepad or WordPad.
You will be able to see associated project information, along with the project GUID, source control information, and other meta data. For whatever unexplained reason, my .sln file only had the details for a single project in the solution, and therefore was being the only one opened up in VS.NET. I didn’t want to start adding projects manually via the IDE thinking that could cause a mess. The solution lies in correcting or replacing the .sln file.
I chose to go to my source control provider and get a 2nd most recent version of the .sln and placed it in a separate location from the real .sln file to inspect. Upon opening the version from source control, I found the details for all (3) projects as expected. There are (2) ways to fix the issue at hand now, and I recommend making a backup of everything before making any modifications to this file in case there is an issue.
I chose to copy everything out of the properly formatted .sln and paste it into the corrupted .sln file and then reopen the solution. The other option would be to simply replace the .sln file directly.
After reopening VS.NET, I didn't use any quick links on the home page and manually navigated back to the .sln file to open the solution. I received one warning/option box stating what was loaded was not the same as in Source Control (because I had modified the .sln manually), and told me it would notify me of issues or differences, which there were not any. I had to reset the startup project and startup .aspx page and all was back to normal.
Monday, August 30, 2010
Sunday, August 22, 2010
How To: Extract Selected Items From An ASP.NET ListBox Using LINQ
(Note: All code examples here use VS.NET 2010 so there is a lot of shortcutting. Just know you will need line continuation underscores "_" and long hand properties if using an earlier version of VS.NET)
If you have wanted to extract all of the 'Selected' items from a ListBox in the past, you probably created a For Each loop similar to the code below:
So to begin let's create a simple ListBox with some colors:
If you have wanted to extract all of the 'Selected' items from a ListBox in the past, you probably created a For Each loop similar to the code below:
However thank you to LINQ and being that the ListBox control is an enumerable type, you can run a LINQ query against the Listbox's Items to get back all of the selected items. One important note to keep in mind is how powerful LINQ is and how many different objects and types can be used in LINQ queries. This example is specific to an ASP.NET ListBox, but there are many other controls where you could write similar queries to extract out data based on a criteria.
For Each li As ListItem In Me.ListBox1.Items
If li.Selected Then
'Code here to process selected item
End If
Next
So to begin let's create a simple ListBox with some colors:
Now on the button click event we want to extract these colors into our class named 'ColorData'. It has (2) properties: ColorID and ColorDesc. So 1st let's just view the simple ColorData class:
<asp:ListBox ID="ListBox1" runat="server" SelectionMode="Multiple">
<asp:ListItem Value="1" Text="Green" />
<asp:ListItem Value="2" Text="Red" />
<asp:ListItem Value="3" Text="Blue" />
<asp:ListItem Value="4" Text="Orange" />
</asp:ListBox>
Like mentioned before, we would have iterated through each ListItem Object in the ListBox control, and manually added each set of values to a generic list. With the LINQ query we can do it all in 1 step. Below is the LINQ query that dumps into an Anonymous type named 'myColorData' the results of the query which places only the selected ListBox item values into the collection:
Public Class ColorData
Public Property ColorID As Integer
Public Property ColorDesc As String
End Class
That's it! Of course you could modify the values inline, check to make sure the values were of the correct type, or anything else required. Hopefully this will get you thinking about getting rid of those old For Each loops when accessing data from ASP.NET controls and using LINQ instead.
Protected Sub Button1_Click(ByVal sender As Object, ByVal e As EventArgs) Handles Button1.Click
'Extract the 'Selected' ListBox items into a generic list of type 'ColorData'
'Use LINQ to extract the items rather than iterating through manually with a For-Each loop
'Also notice the use of an Anonomous type: myColorData which at runtime is resolved to
'a generic list of type ColorData
Dim myColorData = (From li As ListItem In Me.ListBox1.Items
Where li.Selected = True
Select New ColorData With {
.ColorDesc = li.Text,
.ColorID = li.Value})
End Sub
Tuesday, August 3, 2010
Visual Studio LightSwitch Announced
I don't normally just add links to other sites here, but this is a new tool that was announced at the VSLive! conference in Redmond today. Microsoft Visual Studio LightSwitch seems like a useful RAD tool reminiscent a tad of Microsoft Access with wizardry style ability to create data and forms, but with a managed code (.NET Framework) backend, and options for Application Type (desktop or web). Take a look at the link below to learn more:
Introducing Microsoft Visual Studio LightSwitch
Visual Studio LightSwitch 2010 Microsoft® Visual Studio®
Introducing Microsoft Visual Studio LightSwitch
Visual Studio LightSwitch 2010 Microsoft® Visual Studio®
Sunday, August 1, 2010
How To: Turn Off User Account Control (UAC) Prompts for Administrators on Windows Server 2008
There is more than meets the eye when it comes to the User Account Control (UAC) settings configured on the various Microsoft Operating System platforms, and I will not get into it all. I do think it is an important security feature to alert users when accessing an application that has prompted for elevated rights. When this occurs a UAC popup window appears prior to the application launching. This gives the user the chance to cancel any process that was not initiated by them directly.
With that said, if you are an administrator and work either directly or remotely with Windows Server 2008, you may find these pop-ups annoying every time you open IIS Manager, SQL Manager, or another application needing elevated privileges. If you have an understanding of UAC and wish to turn off the pop-ups for Administrators logged on you can do the following:
1. In Windows Server 2008, go to Start -> Administrative Tools -> Local Security Policy.
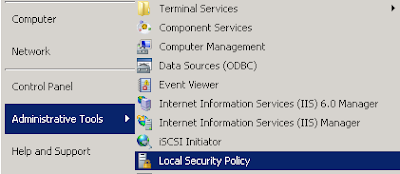
2. In the tree within the Local Security Policy MMC, expand Local Policies -> Security Options.
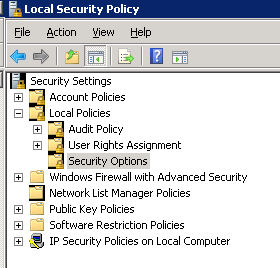
3. Scroll down in alphabetical order on the right-hand side. There are a block of settings for UAC. Again as mentioned before there is more than meets the eye with UAC, but for this operation we are only concerned with a single setting. Find the setting marked 'User Account Control: Behavior of the elevation prompt for Administrators in Admin Approval Mode'. Right-click the setting and select 'Properties'.
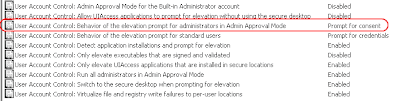
4. Change the dropdown setting to 'Elevate without prompting' and select 'Apply' to close the dialogue. You can also close the Local Policies MMC.
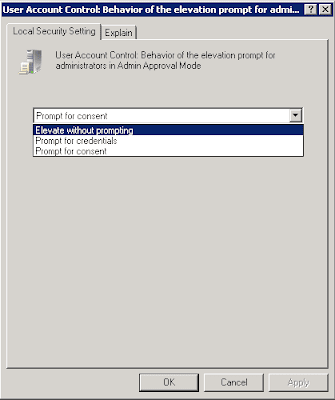
That's all there is to it; now when you open application that requests elevated permissions, you the administrator will not be prompted by any UAC pop-ups.
With that said, if you are an administrator and work either directly or remotely with Windows Server 2008, you may find these pop-ups annoying every time you open IIS Manager, SQL Manager, or another application needing elevated privileges. If you have an understanding of UAC and wish to turn off the pop-ups for Administrators logged on you can do the following:
1. In Windows Server 2008, go to Start -> Administrative Tools -> Local Security Policy.
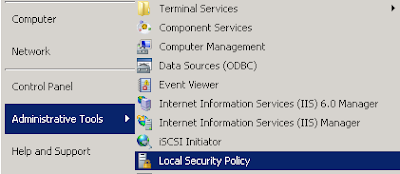
2. In the tree within the Local Security Policy MMC, expand Local Policies -> Security Options.
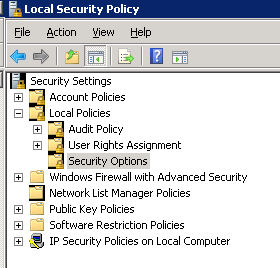
3. Scroll down in alphabetical order on the right-hand side. There are a block of settings for UAC. Again as mentioned before there is more than meets the eye with UAC, but for this operation we are only concerned with a single setting. Find the setting marked 'User Account Control: Behavior of the elevation prompt for Administrators in Admin Approval Mode'. Right-click the setting and select 'Properties'.
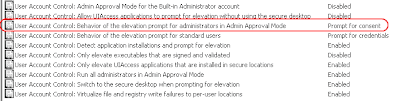
4. Change the dropdown setting to 'Elevate without prompting' and select 'Apply' to close the dialogue. You can also close the Local Policies MMC.
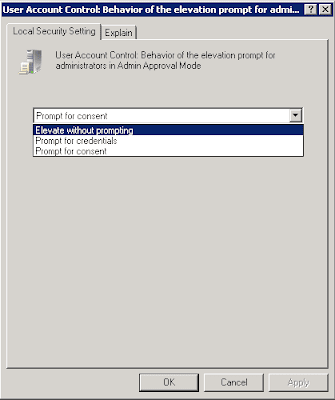
That's all there is to it; now when you open application that requests elevated permissions, you the administrator will not be prompted by any UAC pop-ups.
Subscribe to:
Posts (Atom)